Okay, so I wanted to mess around with time in Python, you know, like, really get a handle on how it works. I decided to build a simple “time handler” – nothing fancy, just something to play with different time formats and maybe do some basic calculations.
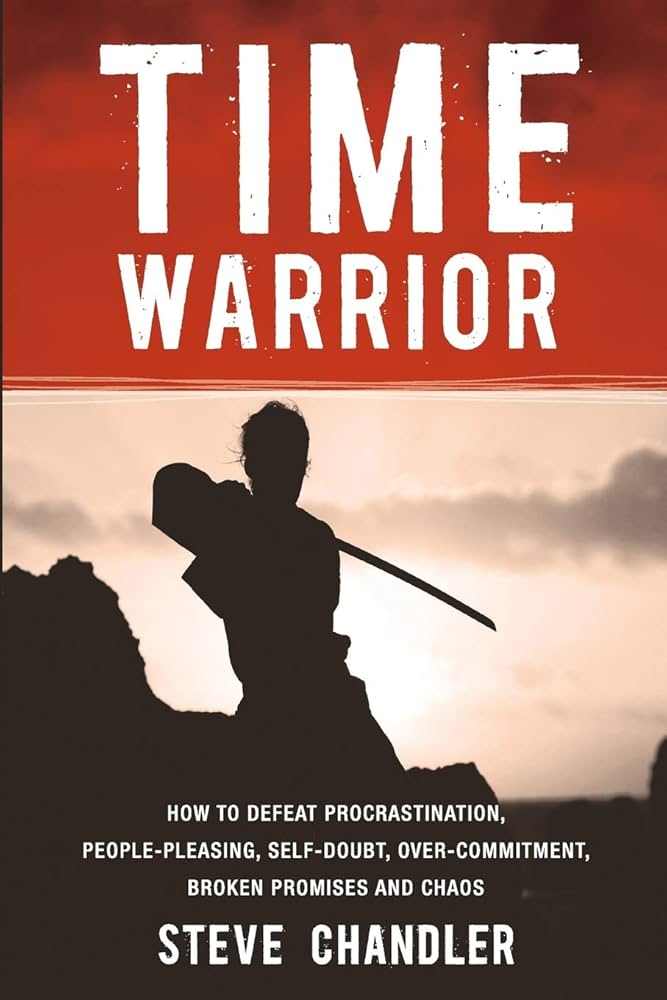
Getting Started
First, I imported the `datetime` module. That’s like, the main thing you need for anything time-related in Python. It’s pretty straightforward.
Then, I created a few basic functions. One was to get the current time, super simple:
- `get_current_time()` – This just used `*()` and returned the current date and time.
I figured I’d want to display the time in different ways, so I made another function:
- `format_time(dt, format_string)`- This one took a `datetime` object (like the one from `get_current_time()`) and a format string. The format string is like a secret code that tells Python how you want the time to look. For example, ‘%Y-%m-%d’ would give you ‘2024-10-27’.
Playing Around
I started playing with this. I called `get_current_time()` and then fed the result into `format_time()` with different format strings. It was pretty cool to see the time displayed in all these different ways.
I then decided to add a function to calculate the difference between two times. I called it.
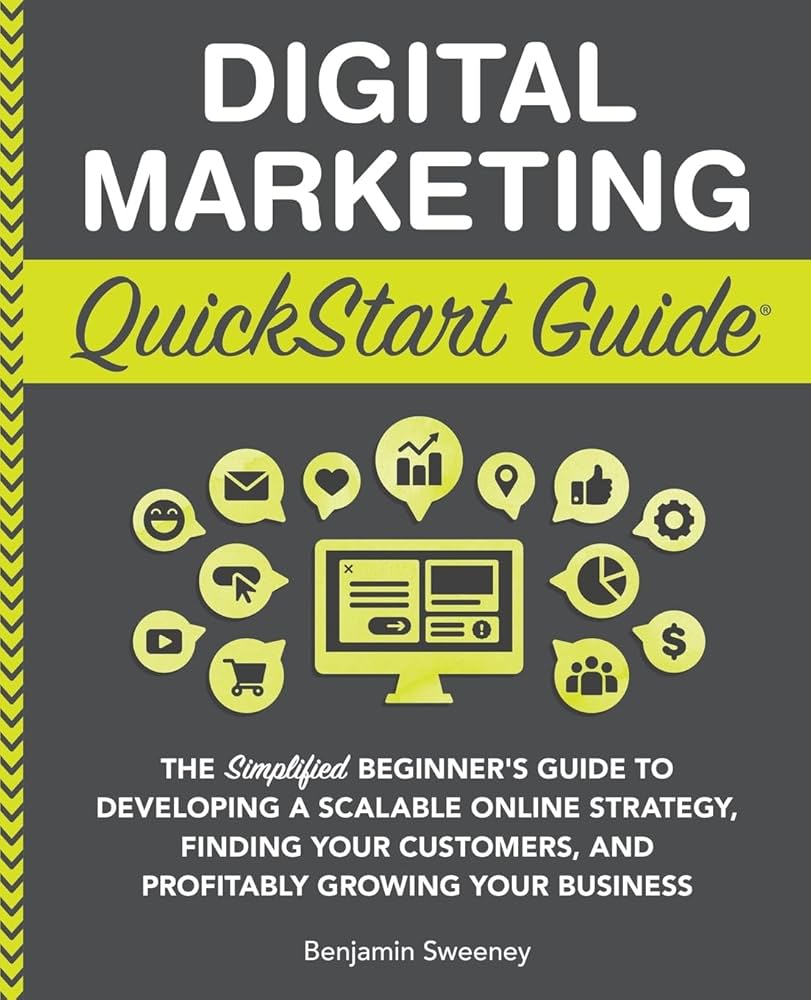
- `time_difference(dt1, dt2)`- This function takes the result from *() and find the difference.
I realized I needed a way to create `datetime` objects from strings, because, you know, sometimes you get time data as text. So I added:
- `parse_time_string(time_string, format_string)` – This is kind of the opposite of `format_time()`. It takes a string and a format string and turns it into a `datetime` object.
Putting It Together
I then combined those functions.
I used `parse_time_string()` to create two `datetime` objects from some made-up time strings. Then I used `time_difference()` to get the difference between them. And finally, I used `format_time()` to display the original times and the difference in a nice, readable way.
It was a bit of trial and error, especially with the format strings. You gotta get those right, or Python gets grumpy. But it was fun! I learned a lot about how to manipulate time in Python, and I have this little tool now that I can use for other projects.
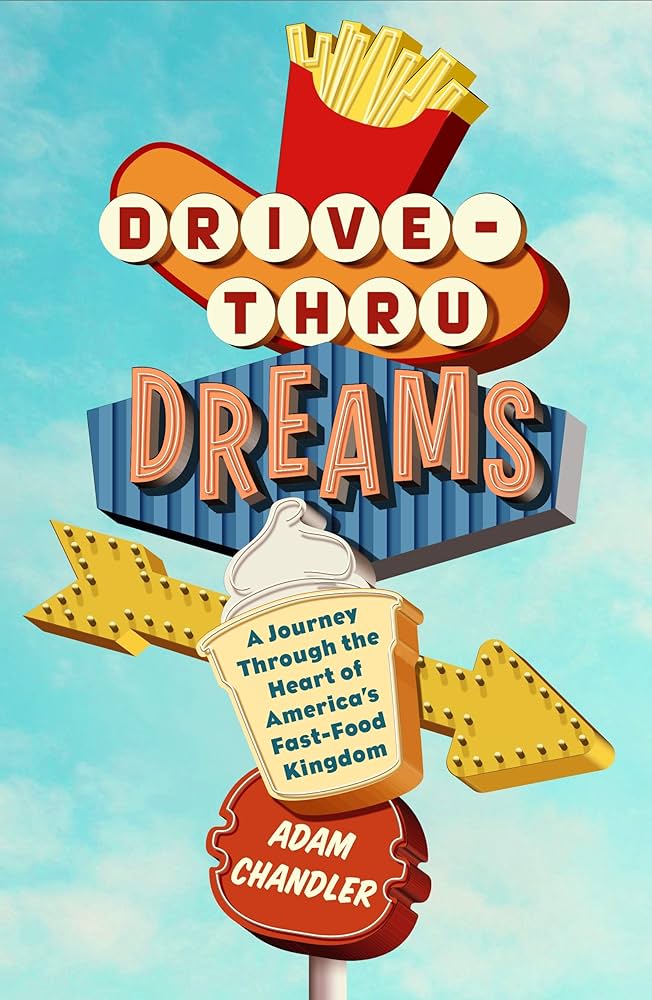
It’s not perfect, but it works! I might add more features later, like handling timezones or something. But for now, it’s a good start.