Alright, so today I’m gonna talk about this little project I messed around with called “cat zoomer.” It’s nothing fancy, just a quick and dirty thing I whipped up, but figured I’d share the process.
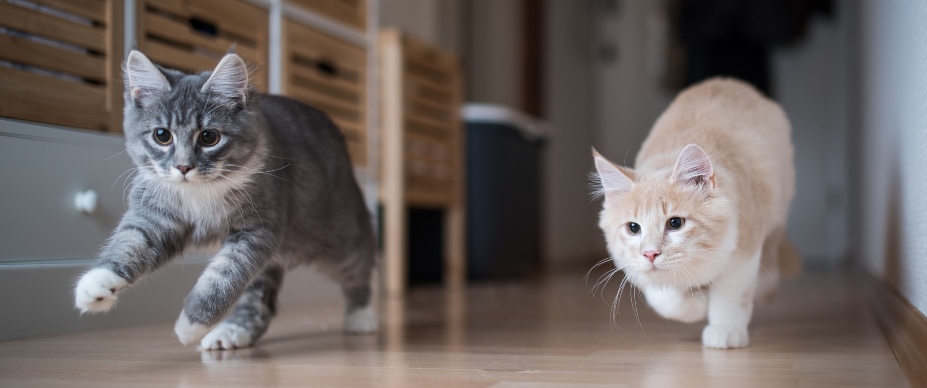
First things first: The idea. I was just goofing off, thinking about those image zooming effects you see all over the web, and thought, “Why not make one that’s ridiculously simple and cat-themed?” I’m a sucker for cats, what can I say?
Next up, the tools. I kept it super basic. Just plain HTML, CSS, and a tiny bit of JavaScript. No frameworks, no libraries, nada. Wanted to keep it pure and see how far I could push it with vanilla code. Plus, it’s good practice, ya know?
Let’s dive into the code!
- HTML: I started with a simple div containing the cat image. Gave it an ID so I could easily grab it with JavaScript.
<div id="cat-container"><img src="*" alt="A cute cat"></div>
Yep, that’s it! Kept it real simple, real clean.
- CSS: This is where I added a bit of styling to make it look decent. I set the container to have a fixed width and height, and used
overflow: hidden
to crop the image when it zoomed. Also, I played around withtransition
to make the zooming smooth.#cat-container {
width: 300px;
height: 200px;
overflow: hidden;
position: relative;
#cat-container img {
width: 100%;
transition: transform 0.3s ease;
#cat-container:hover img {
transform: scale(1.2);
The
:hover
part is what triggers the zoom when you mouse over the image. Pretty straightforward, right? - JavaScript: Okay, this part’s even simpler. I didn’t even need it in the end for the basic zoom. But I was originally planning on adding more complex interaction, so I had this in place:
const catContainer = *('cat-container');
// some other planned stuff.
Basically, just grabbing the container element so I could potentially add some event listeners or something. But for this super basic version, it’s not really doing anything.
The “aha!” moment. The key thing was the CSS transform: scale()
. That’s what actually made the image zoom. And the transition
property made it smooth.
Challenges I ran into: Honestly, not a lot. This was a pretty simple thing. The biggest challenge was probably just tweaking the zoom amount (the scale(1.2)
value) to get it looking just right. I didn’t want it to zoom too much.
What I learned: Even simple effects can be pretty cool! Also, a reminder of how powerful CSS transitions are. You can do a lot with just a few lines of code.
Final thoughts. This “cat zoomer” thing is nothing groundbreaking, but it was a fun little exercise. It’s a good example of how you can take a simple idea and quickly bring it to life with just a few lines of code. Maybe I’ll add some more features to it later, like different zoom levels or the ability to pan the image. Who knows!
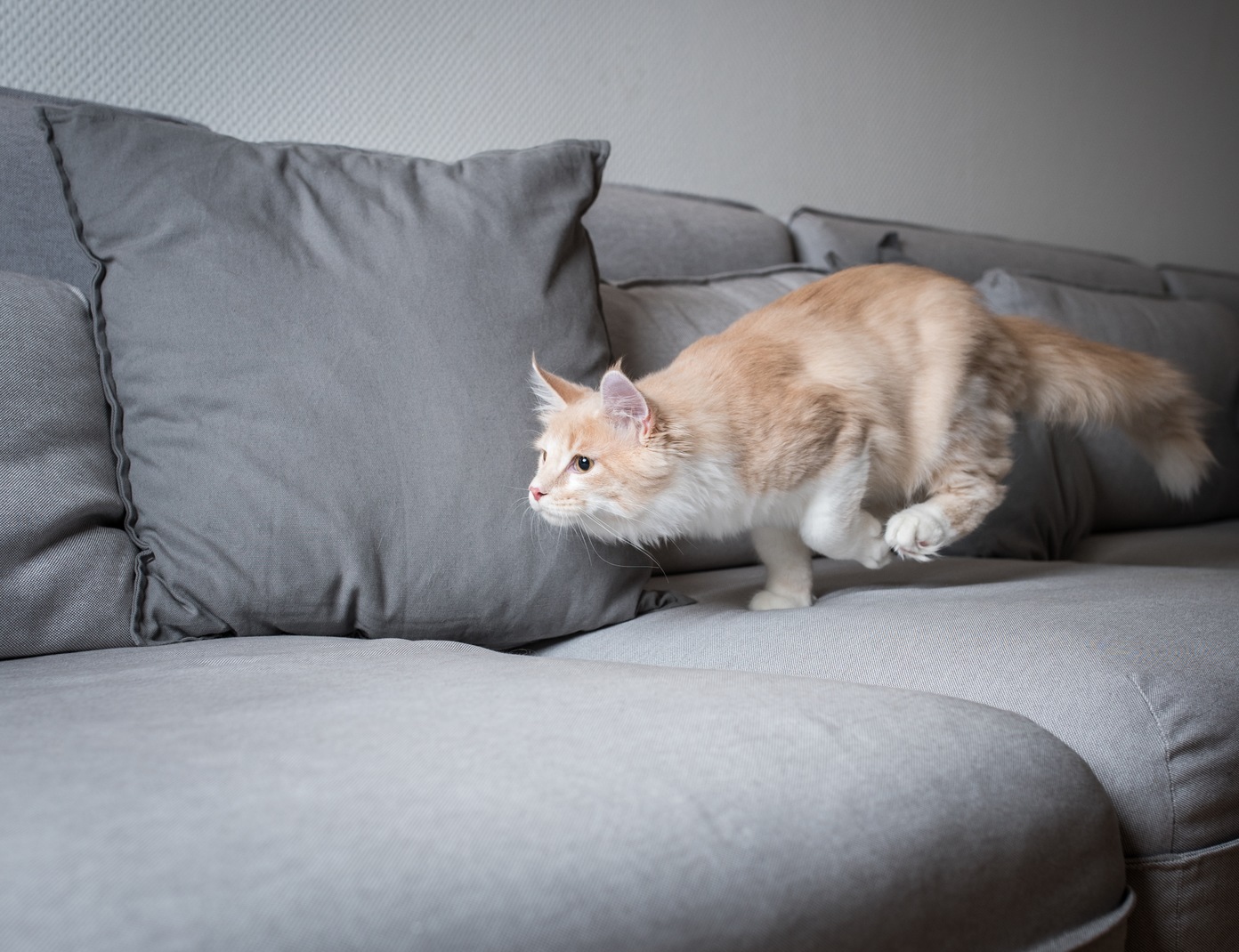
Anyway, that’s the story of my cat zoomer. Hope you found it interesting!