Alright, let’s talk about my little “quick ball” adventure. It’s not as dirty as it sounds, I promise!
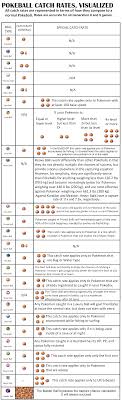
So, I was messing around with some Python code the other day, trying to speed up a process that was taking way too long. I’m talking like, grab-a-coffee-and-watch-paint-dry levels of slow. The task involved a lot of checking if certain items were present in a really long list. Think thousands and thousands of items.
My initial approach was super basic. I just looped through the big list and used the “in” operator to see if my item was there. Something like this:
for item in big_list:
if my_item in big_list:
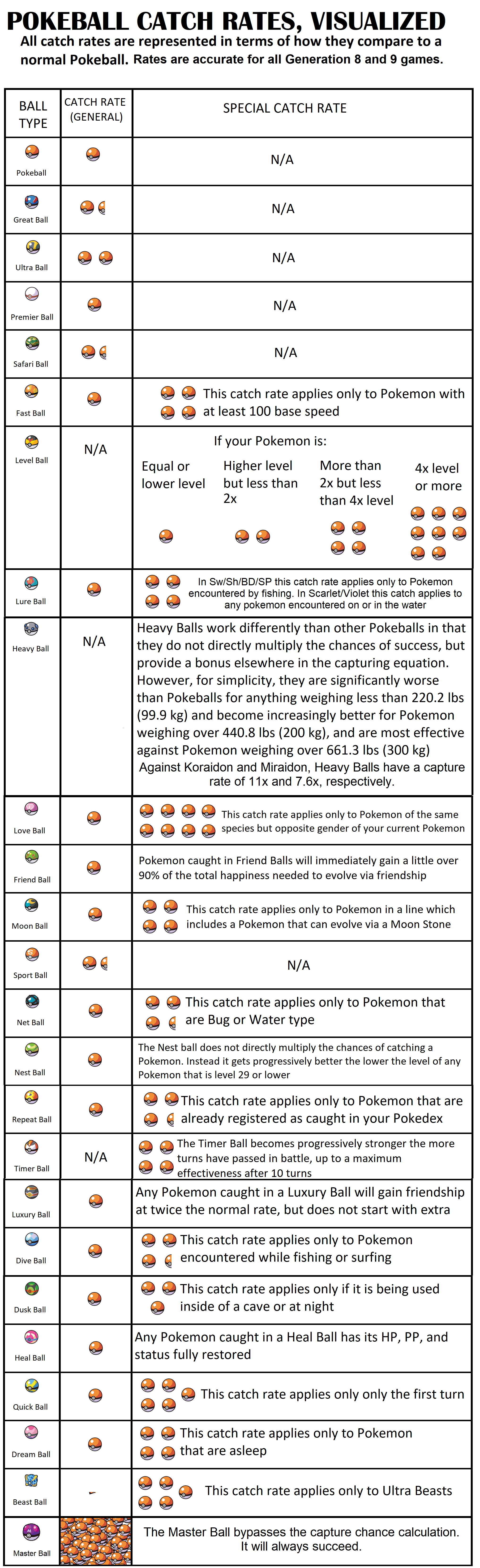
# Do something
Yeah, I know, facepalm material. The problem is that the “in” operator on a list has to go through each item one by one until it finds a match, or it gets to the end. That’s O(n) complexity, which is a fancy way of saying “slow as heck” when n is a huge number.
I remembered something from my old computer science classes: sets! Sets in Python (and many other languages) are implemented using hash tables. This means checking for membership (“is this item in the set?”) is super fast, like O(1) fast. Basically, it’s almost instant, no matter how big the set is.
So, the first thing I did was convert my big list into a set. I just did it like this:
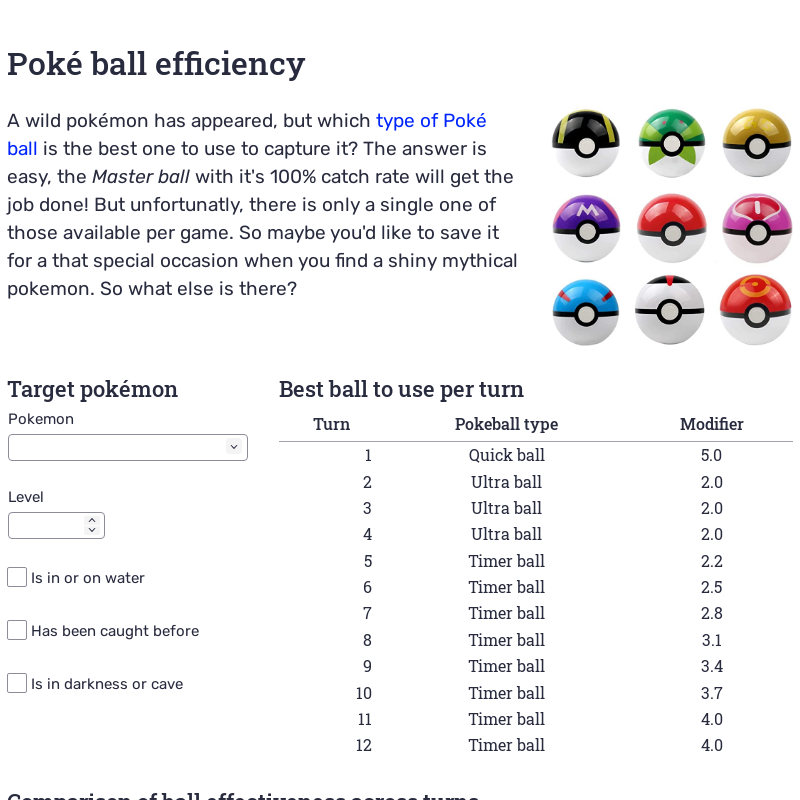
big_set = set(big_list)
Simple enough, right? This creates a new set containing all the items from the original list. Now, instead of using “in” on the list, I could use “in” on the set:
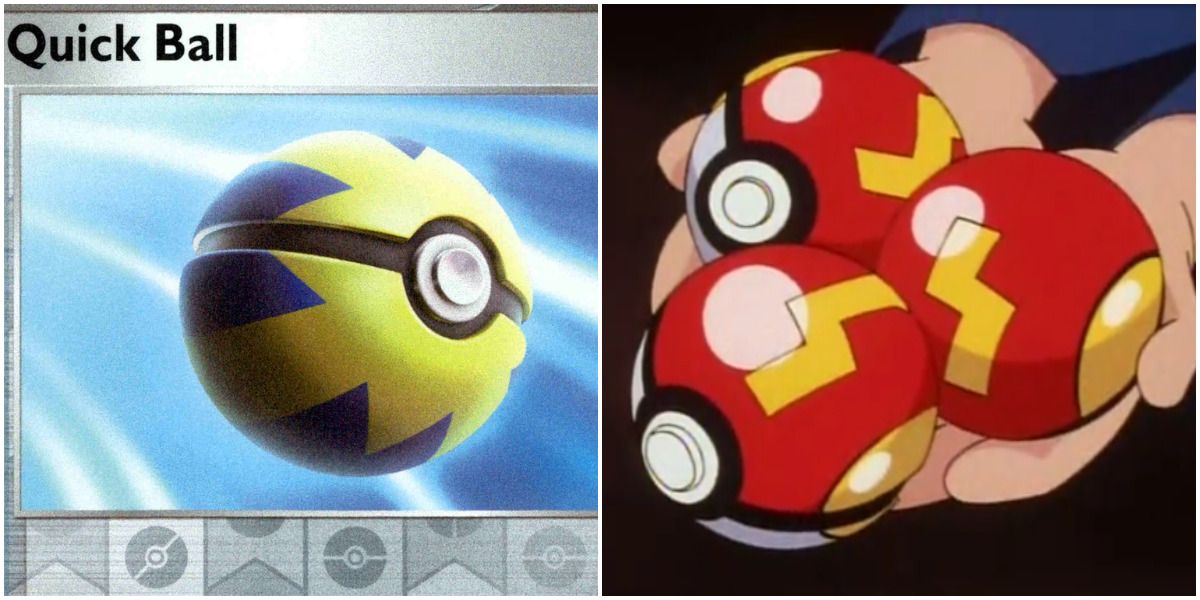
for item in big_list:
if my_item in big_set:
# Do something
The difference was night and day. What used to take several minutes now finished in a fraction of a second. It was like giving the code a shot of adrenaline!
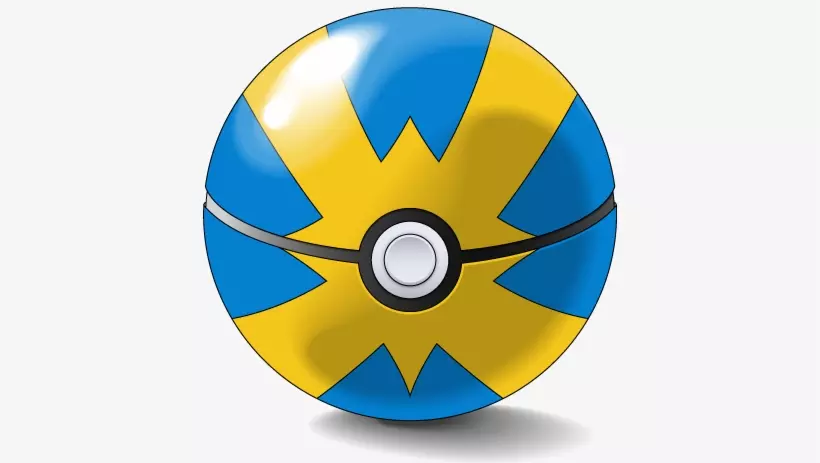
But I didn’t stop there. I thought, “What if I could avoid looping through the entire list at all?”. I realized I could rewrite the logic to only check the items that needed to be checked. This involved a bit more restructuring of my code, but it was worth it.
The final version looked something like this (simplified, of course):
items_to_check = get_items_to_check()
# Function that returns a list of items to check
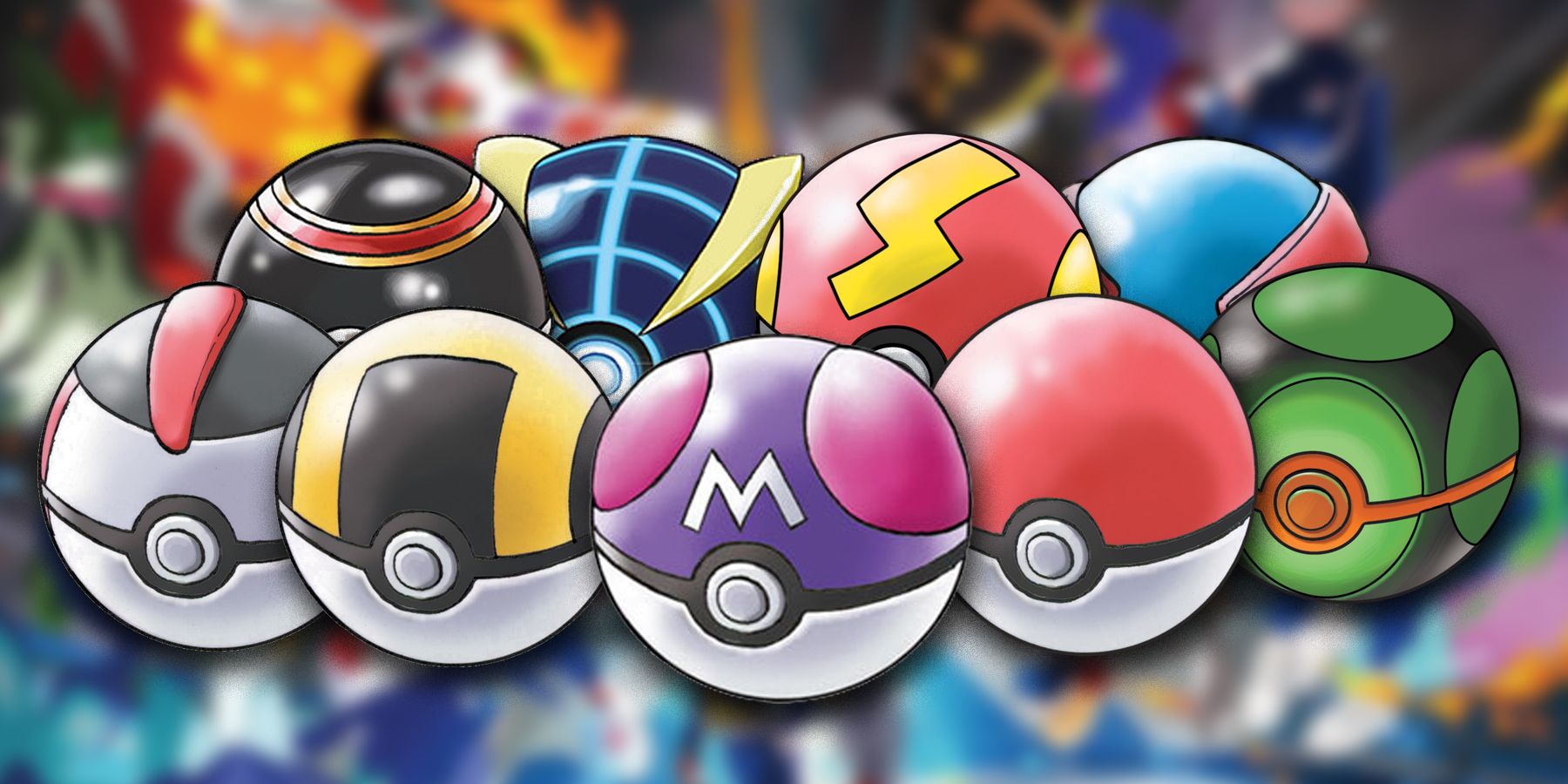
big_set = set(big_list)
for item in items_to_check:
if item in big_set:
# Do something
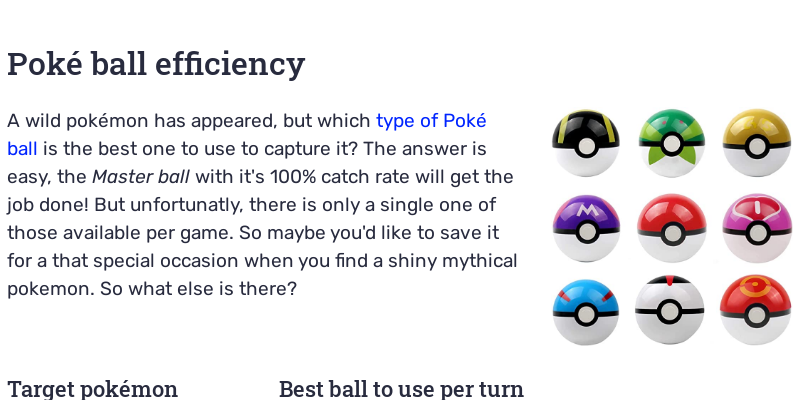
By only checking a small subset of items, and using the set for quick membership checks, I achieved a massive performance boost. I’m talking like, from minutes to milliseconds. That’s the power of picking the right data structure and optimizing your algorithms!
Key Takeaways:
- Lists are slow for membership checking (using “in”).
- Sets are super fast for membership checking.
- Think about your algorithm – can you reduce the amount of data you need to process?
So, that’s my “quick ball” story. It’s a reminder that sometimes the simplest changes can have the biggest impact. And it’s always a good idea to brush up on those data structure basics!