Okay, here’s my attempt at a blog post about my adventures with `curry` and Jordan Poole, keeping the style casual and focused on my hands-on experience.
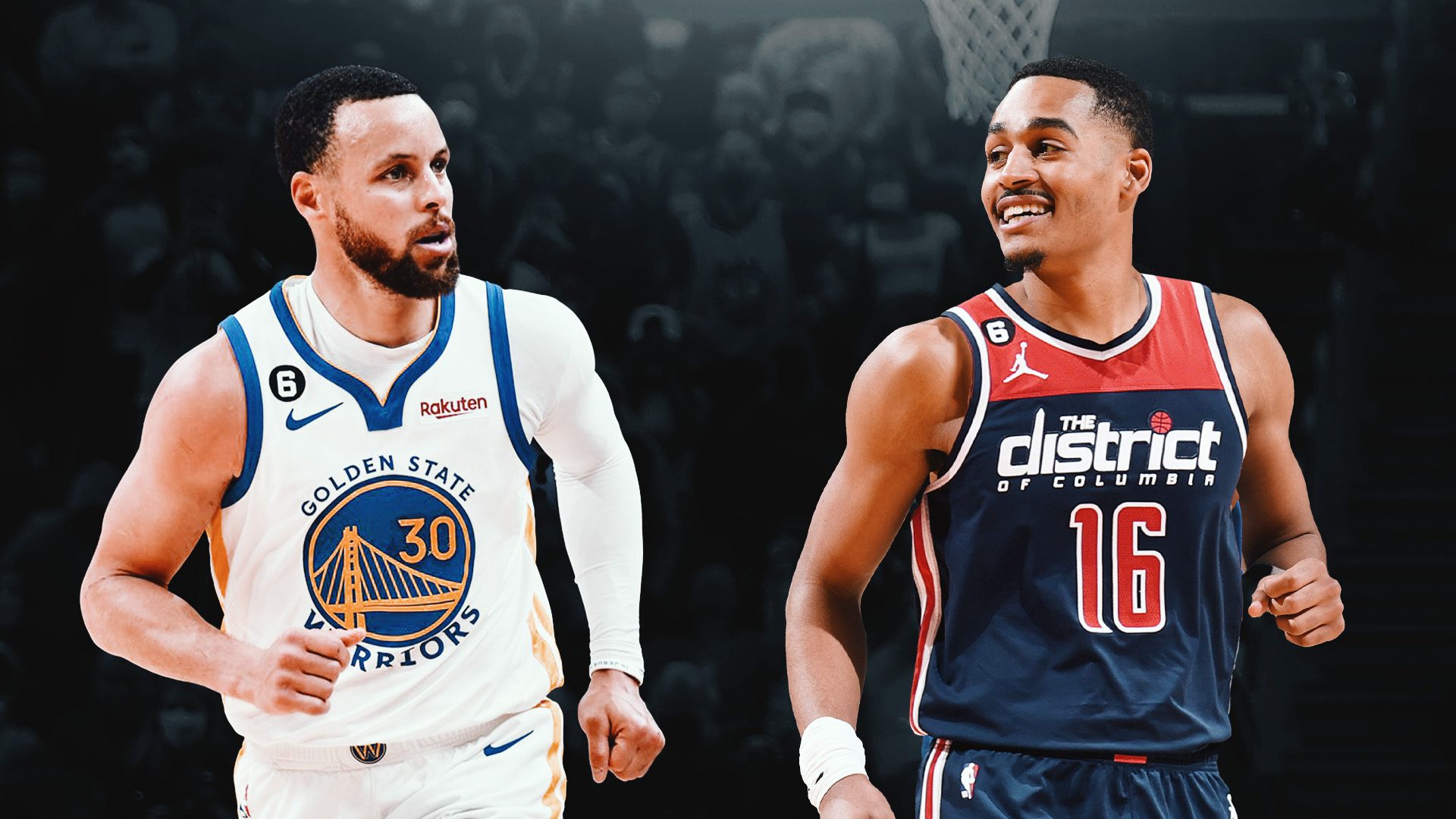
My Curry and Jordan Poole Experiment
Alright folks, so I’ve been messing around with currying in JavaScript, and for some reason, Jordan Poole kept popping into my head. Don’t ask me why. Maybe it’s the handles, maybe it’s the unpredictable nature of both. Anyway, I decided to combine the two.
So, first things first, I needed a problem to solve. I was staring at this piece of code. It was a function that calculated some stuff based on a few arguments like price, tax rate and discount. Super basic.
javascript
function calculateFinalPrice(price, taxRate, discount) {
const taxAmount = price taxRate;
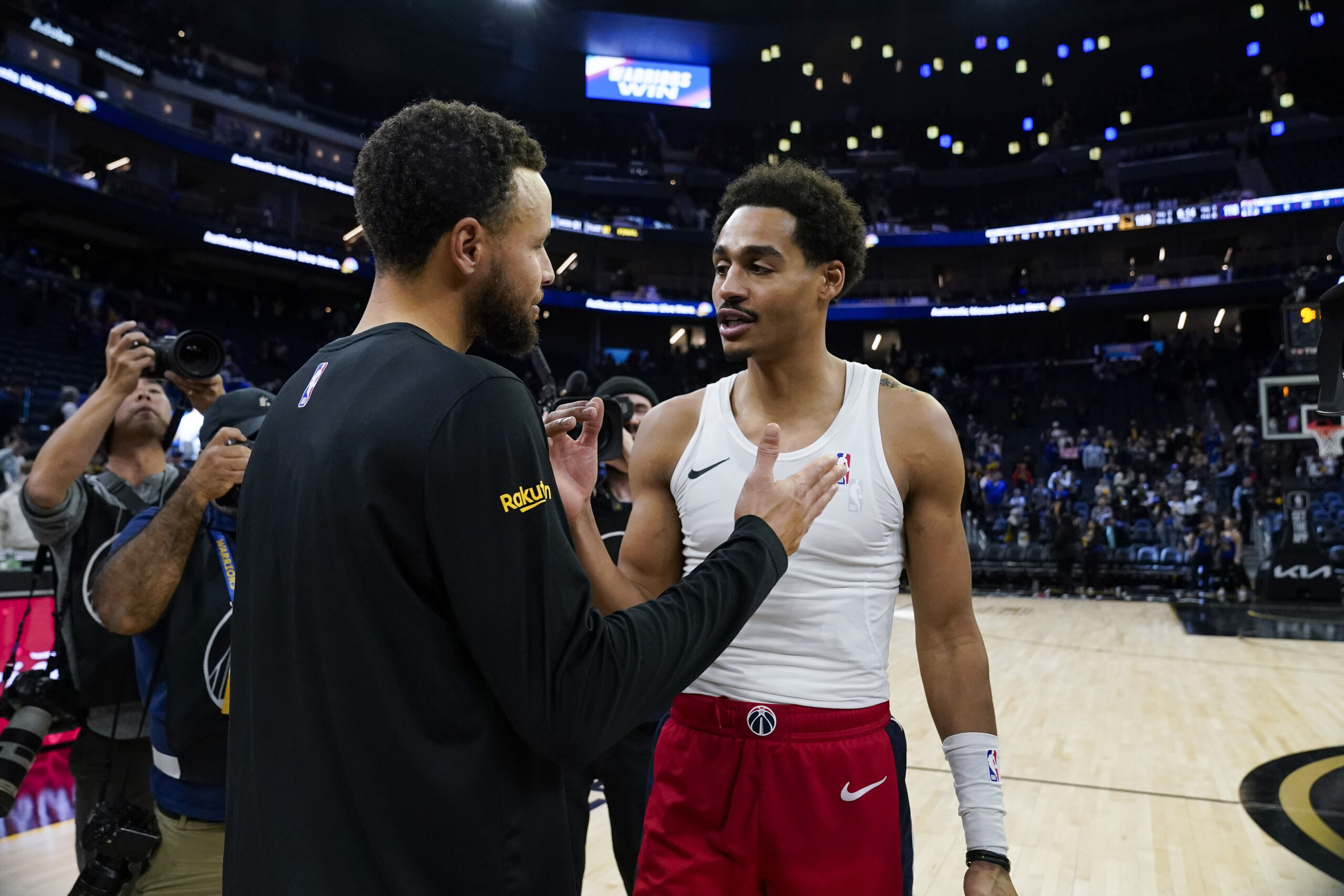
const discountedPrice = price – (price discount);
return discountedPrice + taxAmount;
The thing is, I was always using the same tax rate. Like, always. So, I thought, “Hey, this is ripe for some currying action.”
I started by writing my curry function. There are tons of ways to do this, but I went with a simple one I found online and tweaked a bit. I had to modify the source code, because it had url in the code block.
javascript
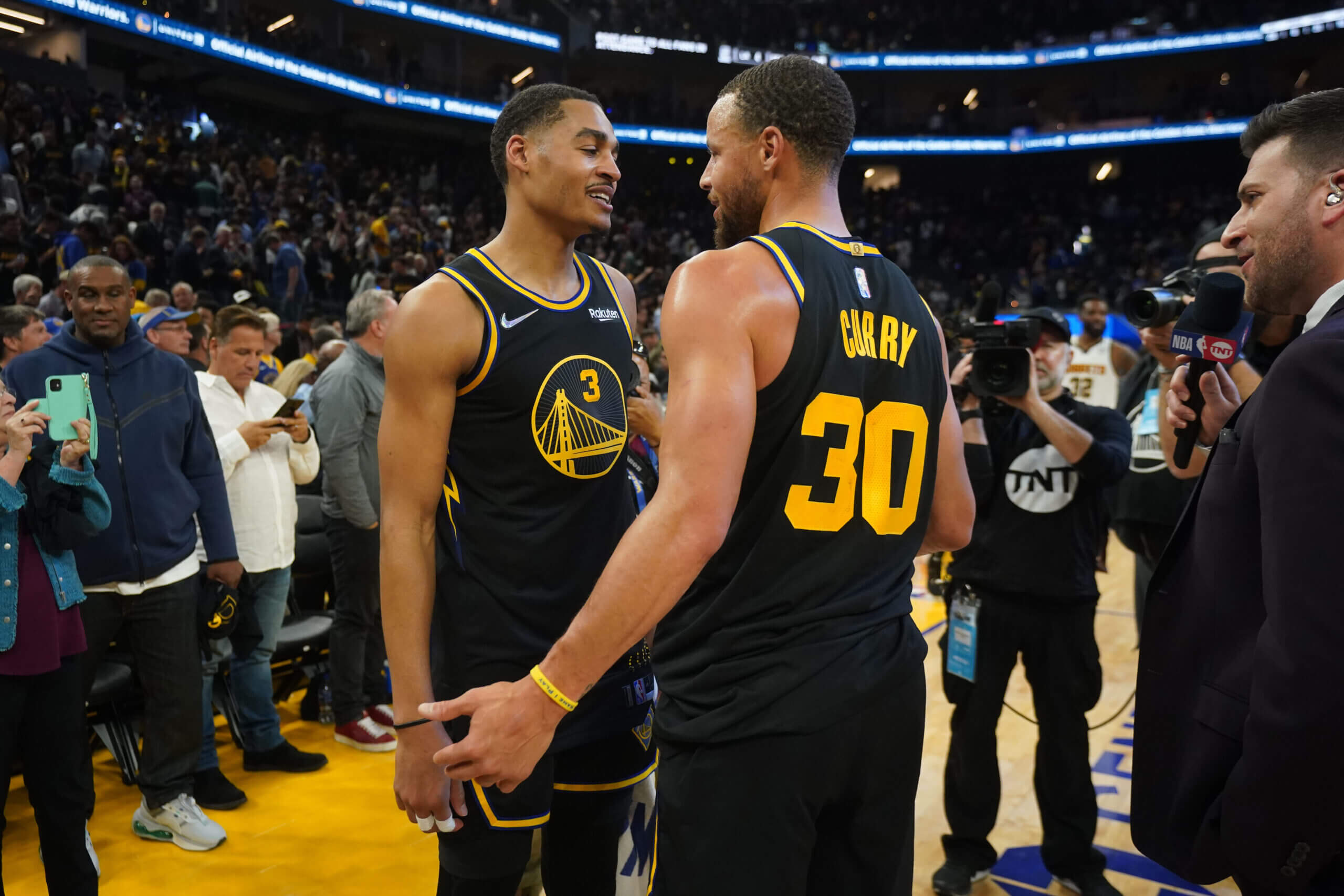
function curry(fn) {
return function curried(…args) {
if (* >= *) {
return *(this, args);
} else {
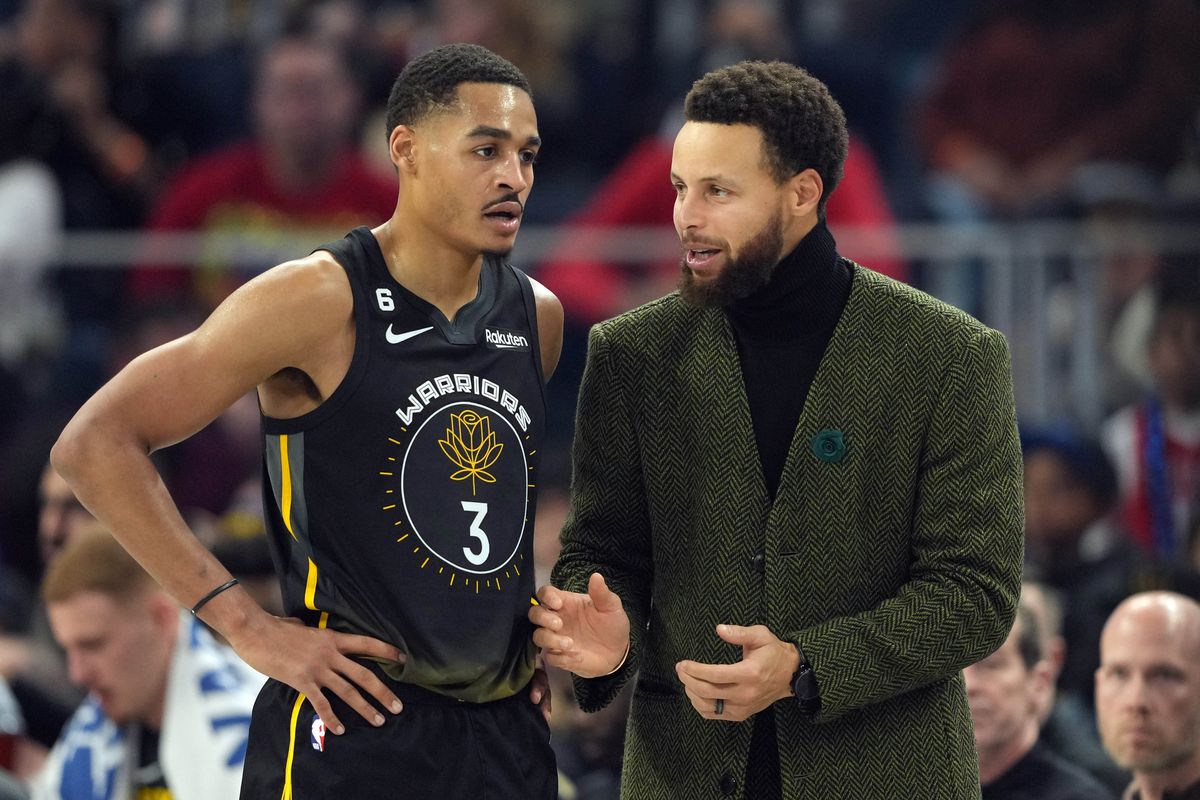
return function(…args2) {
return *(this, *(args2));
Yeah, it’s kinda dense. Basically, it checks if you’ve passed in all the arguments the original function needs. If not, it returns another function that waits for the rest. Keep doing this until you have all the arguments, then boom, runs the original function.
Next, I curried my `calculateFinalPrice` function:
javascript
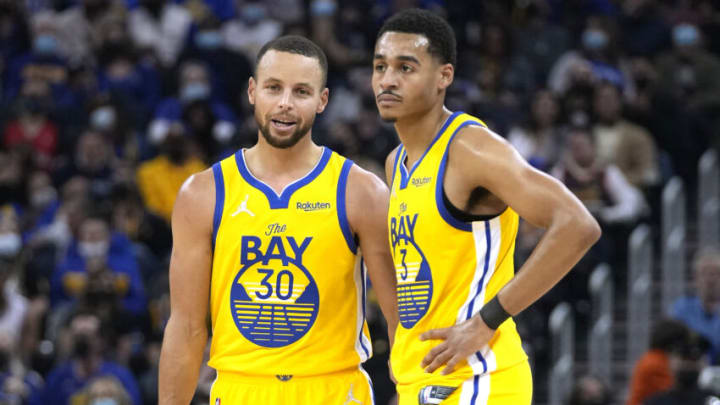
const calculatePriceWithTax = curry(calculateFinalPrice);
Now, the fun part. I could create a specialized function with a fixed tax rate of, say, 0.08 (because that’s the local tax rate, baby):
javascript
const calculatePriceWith8PercentTax = calculatePriceWithTax(undefined, 0.08); // Tax Rate is set
You maybe thinking that it looks weired that the first argument is undefined. Yes, that is true because our first curry function is the price which will be set when we have discount value. It’s kinda weired right, yeah that’s JavaScript.
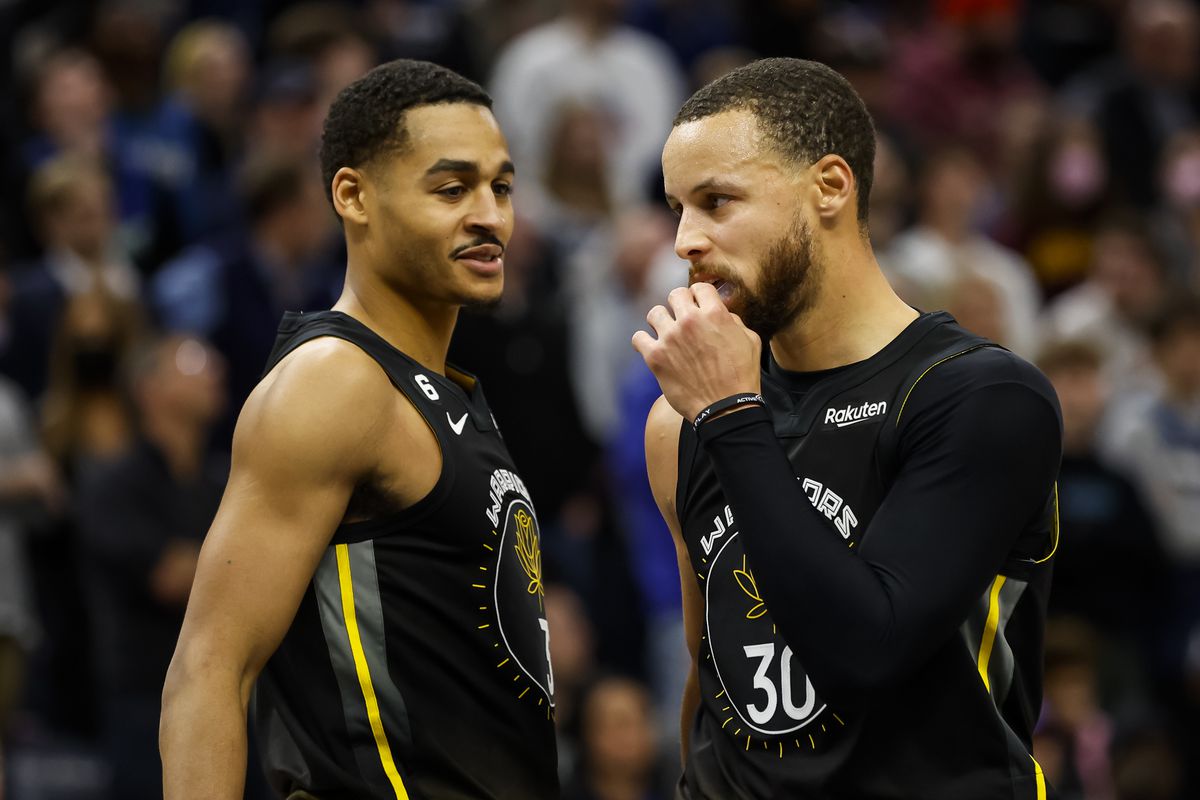
Then, I can do something like:
javascript
const priceAfterTaxAndDiscount = calculatePriceWith8PercentTax(100, 0.1); // set the price and 10% discount
*(priceAfterTaxAndDiscount);
Now that I have curry function ready. I need to somehow relate it to Jordan Poole. So I started to think how could Jordan Poole be part of a function. It’s kinda weired right, but let me tell you what I did.
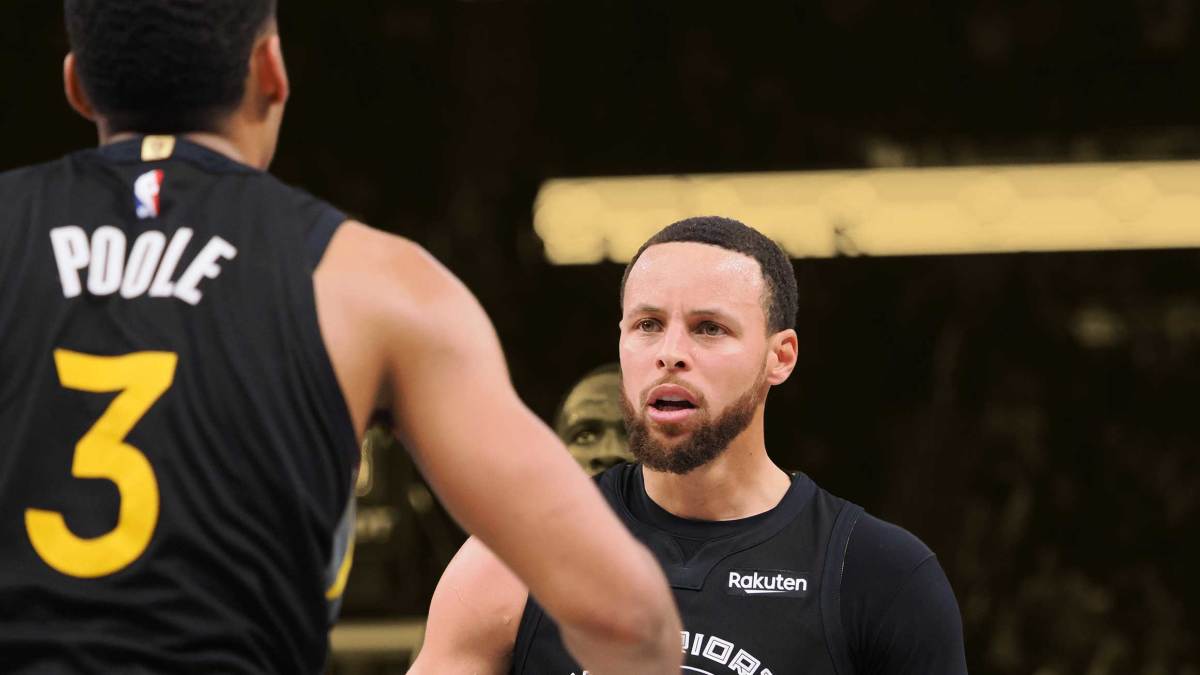
First, I created a JavaScript Object. The object has a property called name and points to string “Jordan Poole”. Then it has a function called “shoot”. The shoot function accepts “successRate” as a parameter.
javascript
const jordanPoole = {
name: “Jordan Poole”,
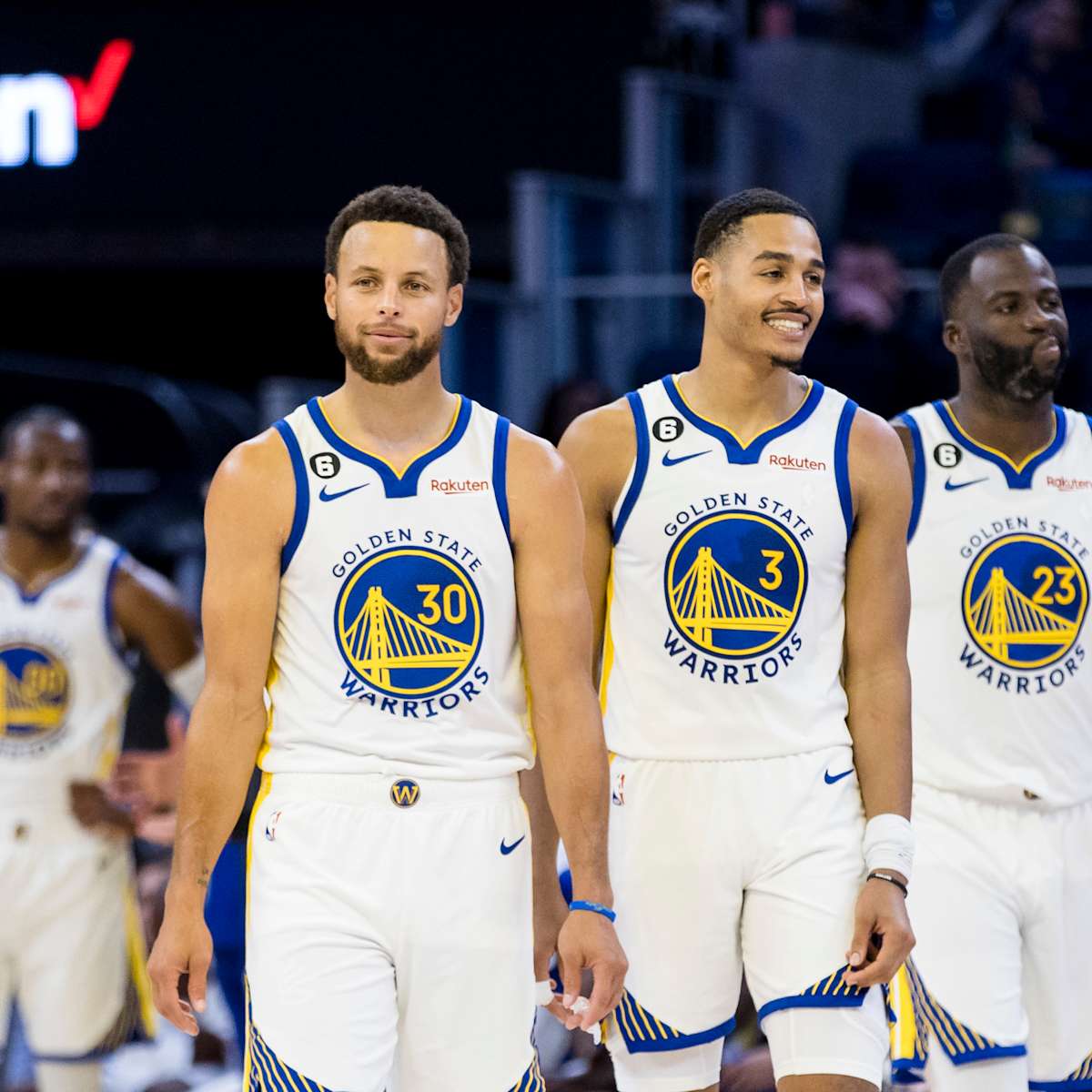
shoot: function(successRate) {
return “Jordan Poole makes ” + successRate + “% of his shots.”;
Okay, I need to somehow combine these two function to make it more interesting, So I think of another use case.
Imagine you’re building a shooting percentage calculator for basketball players. Each player has their own base shooting percentage, but factors like fatigue and defensive pressure can affect their performance. So you need a way to create specialized percentage calculators based on these factors.
javascript
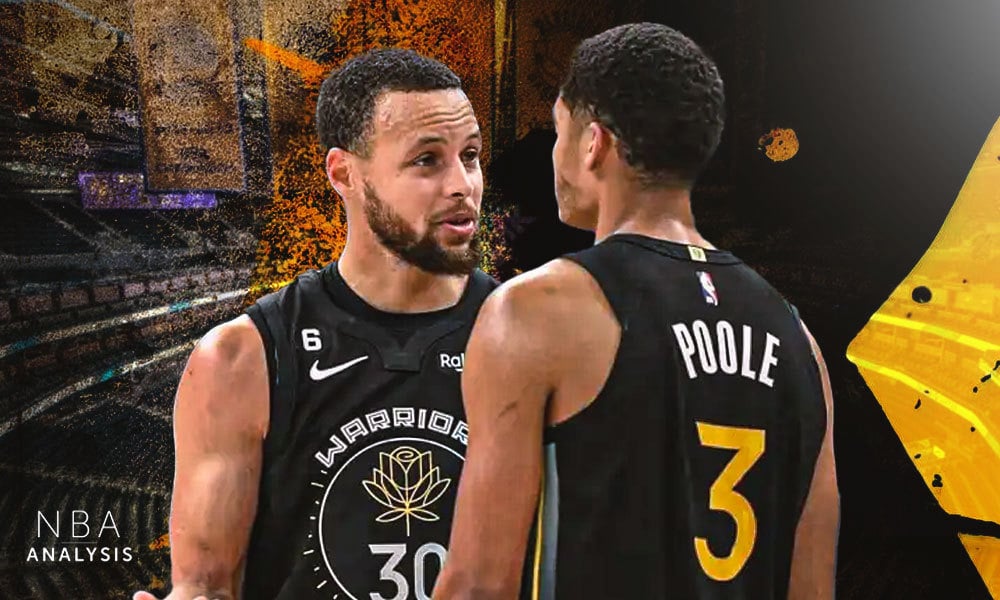
function calculateShootingPercentage(basePercentage, fatigueFactor, defenseFactor) {
return basePercentage fatigueFactor defenseFactor;
Let’s curry the function first
javascript
const calculatePlayerPercentage = curry(calculateShootingPercentage);
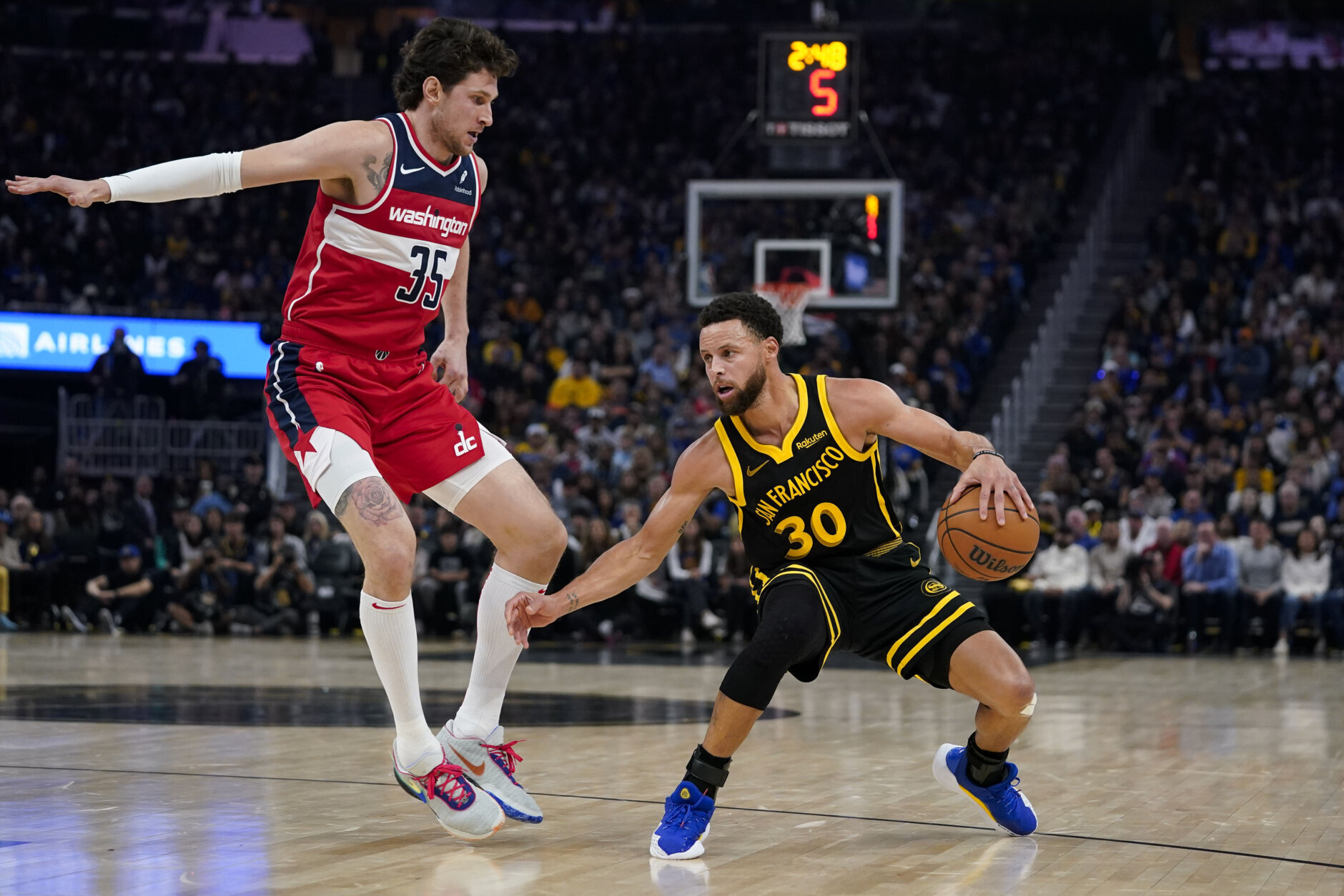
Assuming the player is Jordan Poole and we set the fatigueFactor to 0.9 and defenseFactor to 0.8
javascript
const calculateJordanPoolePercentage = calculatePlayerPercentage(0.445, 0.9, 0.8);
This give us the shooting percentage. Then we can put it inside the object as well!
javascript
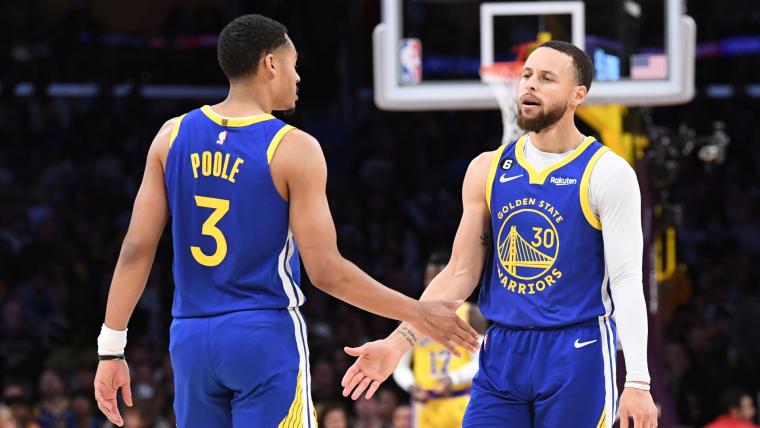
const jordanPoole = {
name: “Jordan Poole”,
calculatePercentage: calculatePlayerPercentage(0.445) // basePercentage known
const percentageWithFatigue = *(0.9);
const finalPercentage = percentageWithFatigue(0.8);
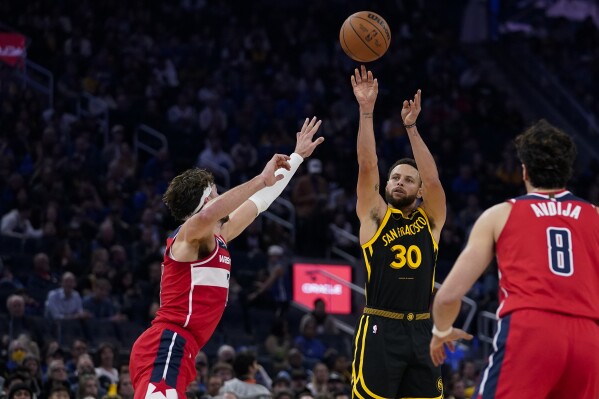
*(“Jordan Poole’s final percentage is: ” + finalPercentage);
Now, this all might seem like overkill for such a simple example. But think about it. When you have a complex application with a lot of configuration or dependencies, currying can be a powerful way to create reusable, specialized functions. It can make your code cleaner, more readable, and easier to test.
So, what did I learn? Currying is cool. Jordan Poole is… well, Jordan Poole. And sometimes, the most random combinations can lead to interesting results. Now I’m off to curry all the things! Maybe I’ll try combining it with generative AI next. Who knows?